This post presents a subclass of UITextField
with custom text padding. Following the method in this post will allow you to change tthe spacing between the frame of a UITextField
and the contained text.
Subclass UITextField
The first step is to subclass UITextField
and define the desired text padding.
class TextFieldWithPadding: UITextField {
var textPadding = UIEdgeInsets(
top: 10,
left: 20,
bottom: 10,
right: 20
)
}
Override textRect(forBounds:) and editingRect(forBounds:)
The next step is to override textRect(forBounds:) -> CGRect
and editingRect(forBounds:) -> CGRect
. Use textRect(bounds:) -> CGRect
to return the drawable area for the text in a UITextField
. Use editingRect(forBounds:) -> CGRect
to return the area in the UITextField
where editable text can be displayed.
class TextFieldWithPadding: UITextField {
var textPadding = UIEdgeInsets(
top: 10,
left: 20,
bottom: 10,
right: 20
)
override func textRect(forBounds bounds: CGRect) -> CGRect {
let rect = super.textRect(forBounds: bounds)
return rect.inset(by: textPadding)
}
override func editingRect(forBounds bounds: CGRect) -> CGRect {
let rect = super.editingRect(forBounds: bounds)
return rect.inset(by: textPadding)
}
}
UITextField With Text Padding
That’s it! With the TextFieldWithPadding
implementation you can achieve the following results:
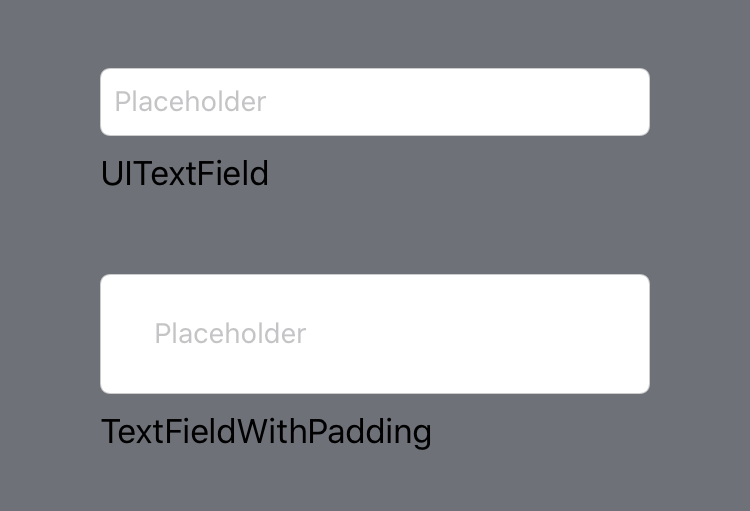