This post presents an extension of UIColor
supporting lighter and darker variants of a UIColor
. Following the method in this post will allow you to generate lighter or darker variants of a UIColor
on demand.
Get Slightly Lighter and Darker Color Variations
The first step is to extract red, green, blue, and alpha components from the current UIColor
. Then, to each color component, add a componentDelta
to make the color lighter or darker. Each color component value is between 0
and 1
.
extension UIColor {
private func makeColor(componentDelta: CGFloat) -> UIColor {
var red: CGFloat = 0
var blue: CGFloat = 0
var green: CGFloat = 0
var alpha: CGFloat = 0
// Extract r,g,b,a components from the
// current UIColor
getRed(
&red,
green: &green,
blue: &blue,
alpha: &alpha
)
// Create a new UIColor modifying each component
// by componentDelta, making the new UIColor either
// lighter or darker.
return UIColor(
red: add(componentDelta, toComponent: red),
green: add(componentDelta, toComponent: green),
blue: add(componentDelta, toComponent: blue),
alpha: alpha
)
}
}
Safely Add RGBA Component Values
Each color component value must be between 0
and 1
. To ensure adding a componentDelta
does not result in a value less than 0
or greater than 1
, use the following function:
extension UIColor {
// Add value to component ensuring the result is
// between 0 and 1
private func add(_ value: CGFloat, toComponent: CGFloat) -> CGFloat {
return max(0, min(1, toComponent + value))
}
}
Make A UIColor Lighter, Darker
The last step is to implement the interface for obtaining a lighter or darker color. Modify the componentDelta
value to control how much lighter or darker the resulting UIColor
is.
extension UIColor {
func lighter(componentDelta: CGFloat = 0.1) -> UIColor {
return makeColor(componentDelta: componentDelta)
}
func darker(componentDelta: CGFloat = 0.1) -> UIColor {
return makeColor(componentDelta: -1*componentDelta)
}
}
UIColor Extension For Slightly Lighter Or Darker Colors
That’s it! With the UIColor
extension you can use lighter and darker UIColor
s on demand, for example:
let redColor = UIColor.red
let lightRed = redColor.lighter()
let darkRed = redColor.darker()
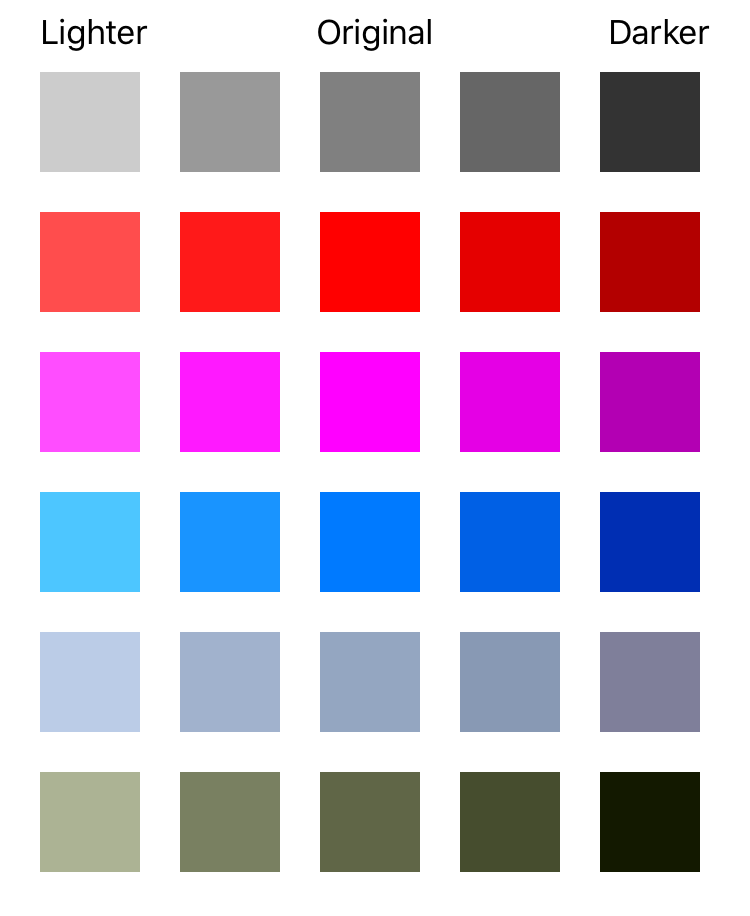