Many apps use location to personalize the app experience for users around the world. This post presents an overview of getting a users location using CLLocationManager
, asking for location permissions, and using CLLocation
in Swift:
- Get A User’s Location Once
- Check Location Permissions Using
CLLocationManager
- Request Location Permissions
a. Location Always and When In Use Usage Description
b. Location When In Use Usage Description - Get Real-Time User Location Updates
CLLocation
, Longitude, and Latitude
Get A User’s Location Once
Sometimes an app only needs a user’s location once, or at one-off times. Only once location authorization can be requested by using the CLLocationManager
method requestLocation()
.
import CoreLocation
// Create a CLLocationManager and assign a delegate
let locationManager = CLLocationManager()
locationManager.delegate = self
// Request a user’s location once
locationManager.requestLocation()
Implement the CLLocationManagerDelegate
method locationManager(:, didUpdateLocations:)
to handle the requested user location. This method will be called once after using locationManager.requestLocation()
.
func locationManager(
_ manager: CLLocationManager,
didUpdateLocations locations: [CLLocation]
) {
if let location = locations.first {
let latitude = location.coordinate.latitude
let longitude = location.coordinate.longitude
// Handle location update
}
}
If the location request fails for any reason, the CLLocationManagerDelegate
method locationManager(:, didFailWithError:)
will be called:
func locationManager(
_ manager: CLLocationManager,
didFailWithError error: Error
) {
// Handle failure to get a user’s location
}
Check Location Permissions Using CLLocationManager
The CLLocationManager
method authorizationStatus()
returns the current location permission authorization for getting a user location inside your application.
// Get the current location permissions
let status = CLLocationManager.authorizationStatus()
// Handle each case of location permissions
switch status {
case .authorizedAlways:
// Handle case
case .authorizedWhenInUse:
// Handle case
case .denied:
// Handle case
case .notDetermined:
// Handle case
case .restricted:
// Handle case
}
Request Location Permissions
Location permissions must first be requested to get user location updates in real-time using location services. There are two types of location permissions your app can request:
- Always. Request always location permissions if your app needs location updates all the time, even if the app is in the background.
- When In Use. Request when in use location permissions if your app only needs location updates while the user is using the app.
To prepare to ask the user for location permissions, modify your app’s Info.plist
using one of the following keys:
Location Always and When In Use Usage Description
The string value for “Locations Always and When In Use Usage Description” in the Info.plist
will be displayed to the user when requesting always location permissions.
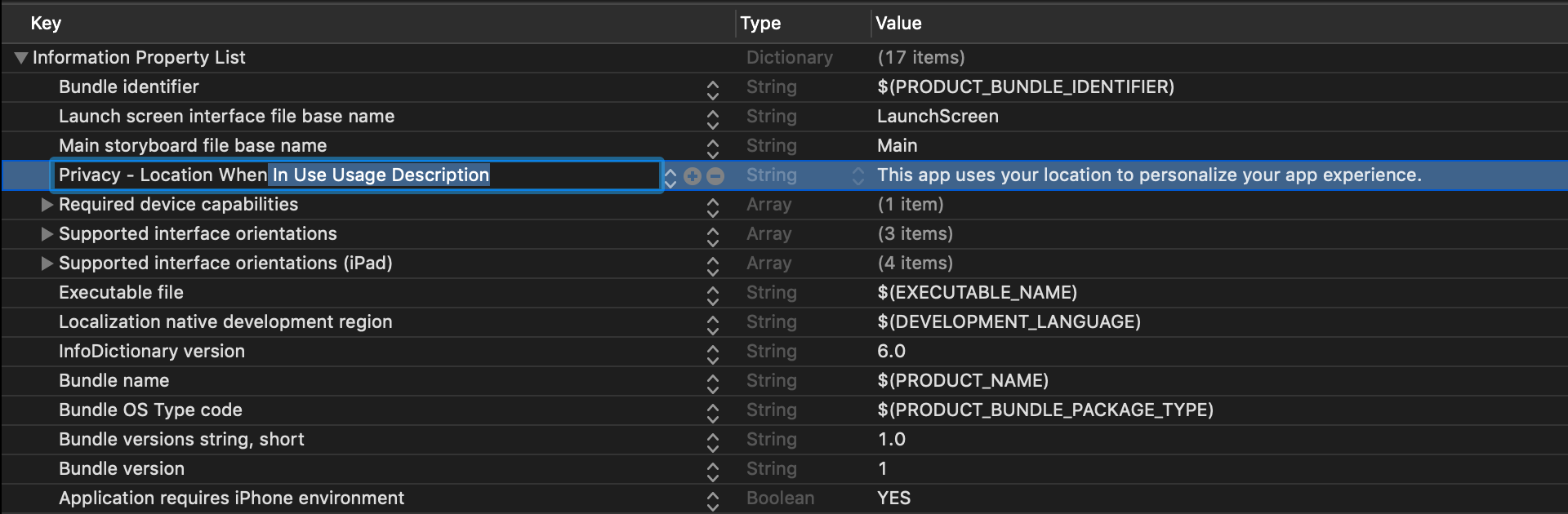
After adding the “Locations Always and When In Use Usage Description” key to the Info.plist
, you can request location permissions:
import CoreLocation
// Create a CLLocationManager and assign a delegate
let locationManager = CLLocationManager()
locationManager.delegate = self
// Use requestAlwaysAuthorization if you need location
// updates even when your app is running in the background
locationManager.requestAlwaysAuthorization()
Location When In Use Usage Description
The string value for “Location When In Use Usage Description” in the Info.plist
will be displayed to the user when requesting when in use location permissions.
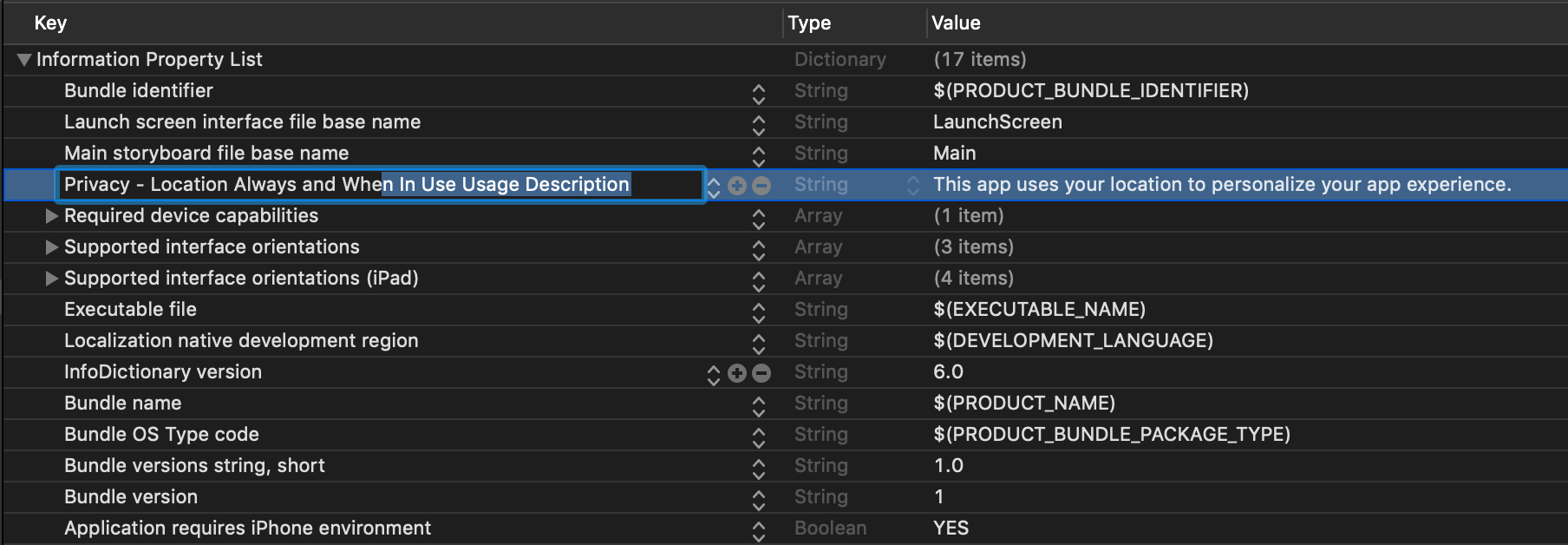
After adding the “Location When In Use Usage Description” key to the Info.plist
, you can request location permissions:
import CoreLocation
// Create a CLLocationManager and assign a delegate
let locationManager = CLLocationManager()
locationManager.delegate = self
// Use requestWhenInUseAuthorization if you only need
// location updates when the user is using your app
locationManager.requestWhenInUseAuthorization()
Get Real-Time User Location Updates
With user permissions, call startUpdatingLocation
on a CLLocationManager
to start location updates.
import CoreLocation
// Create a CLLocationManager and assign a delegate
let locationManager = CLLocationManager()
locationManager.delegate = self
// Start updating location
locationManager.startUpdatingLocation()
// Make sure to stop updating location when your
// app no longer needs location updates
locationManager.stopUpdatingLocation()
Implement the following CLLocationManagerDelegate
methods to handle real-time user location updates:
func locationManager(
_ manager: CLLocationManager,
didChangeAuthorization status: CLAuthorizationStatus
) {
// Handle changes if location permissions
}
func locationManager(
_ manager: CLLocationManager,
didUpdateLocations locations: [CLLocation]
) {
if let location = locations.last {
let latitude = location.coordinate.latitude
let longitude = location.coordinate.longitude
// Handle location update
}
}
func locationManager(
_ manager: CLLocationManager,
didFailWithError error: Error
) {
// Handle failure to get a user’s location
}
CLLocation, Longitude, and Latitude
A location returned by CLLocationManager
is a CLLocation
. Apps frequently get the longitude and latitude of a user’s location from a CLLocation
:
// Given a CLLocation, location
let latitude = location.coordinate.latitude
let longitude = location.coordinate.longitude
Other useful properties of a CLLocation
that you may want to use in your apps are:
// Altitude
let altitude = location.altitude
// Speed
let speed = location.speed
// Course, degrees relative to due north
let source = location.course
// Distance between two CLLocation
// Given another CLLocation, otherLocation
let distance = location.distance(from: otherLocation)
Use Location Services To Get User Location Coordinates In Real-Time
That’s it! By using location services and CLLocationManager
you can request location permissions, check authorization status, get user location updates to personalize your app experience in Swift.