Documentation is an important part of the software development process for iOS and macOS apps. This post presents examples of the different types of comments in Swift, and an example of how to add Quick Help documentation in Xcode:
- Single Line Comments
- Multi-line Comments
- Markdown Comments
a. Comparison of Multi-line and Markdown Comments - Swift Documentation Markup
- Xcode Quick Help Documentation
Single Line Comments
A single line Swift comment is added using a //
prefix:
// Saves changes to the persistent store
func save() throws {
try managedObjectContext.save()
}
Comments can be added at any level of indentation:
func save() throws {
// Saves changes to the persistent store
try managedObjectContext.save()
}
Single line comments can also be added after a line of code:
func save() throws {
try managedObjectContext.save() // Saves changes
}
Multi-line Comments
One way of adding multi-line comments in Swift is the /* ... */
syntax. The /*
starts the multi-line comment, and the */
ends the multi-line comment:
/* Saves changes to the persistent store
if the context has uncommitted changes */
func save() throws {
if managedObjectContext.hasChanges {
try managedObjectContext.save()
}
}
A multi-line comment can also be added as a combination of //
single line comments:
// Saves changes to the persistent store
// if the context has uncommitted changes
func save() throws {
if managedObjectContext.hasChanges {
try managedObjectContext.save()
}
}
Markdown Comments
Xcode will render markdown comments in Swift with markdown styling applied, allowing richer details to be added to Swift documentation. Use ///
to indicate a comment with markdown styling:
/// # Save
/// Saves changes to the persistent store if
/// the context has uncommitted changes
func save() throws {
if managedObjectContext.hasChanges {
try managedObjectContext.save()
}
}
Xcode will also parse comments using /** ... */
syntax as markdown comments. The /**
starts the multi-line markdown comment and the */
ends the multi-line markdown comment:
/**
# Save
Saves changes to the persistent store if
the context has uncommitted changes
*/
func save() throws {
if managedObjectContext.hasChanges {
try managedObjectContext.save()
}
}
Comparison of Multi-line and Markdown Comments
Markdown comment styling allows for richer documentation formatting right in Xcode:
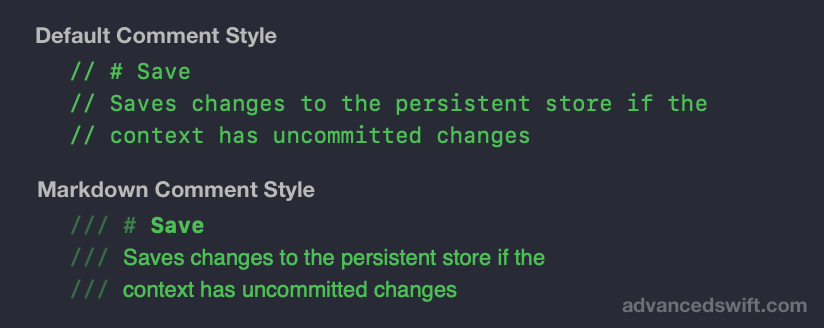
Swift Documentation Markup
The Swift project includes support for documentation comments using Swift Markup. Swift Markup defines a number of special prefixes that are used when generating Swift documentation:
parameter x: Parameter description
is used to describe parametersreturns: Return description
is used to describe a function’s return valuethrows: Condition description
is used to describe under what circumstances a function will throw an error
To learn about all the options available in Swift Markup, check out the Field Extensions section of the Documentation Comment Syntax description in the Swift repository.
Xcode Quick Help Documentation
In Xcode, right-clicking on a function presents a “Show Quick Help”
option. For many types and functions that are part of Apple frameworks, Quick Help displays a popup with useful information about the type or function:
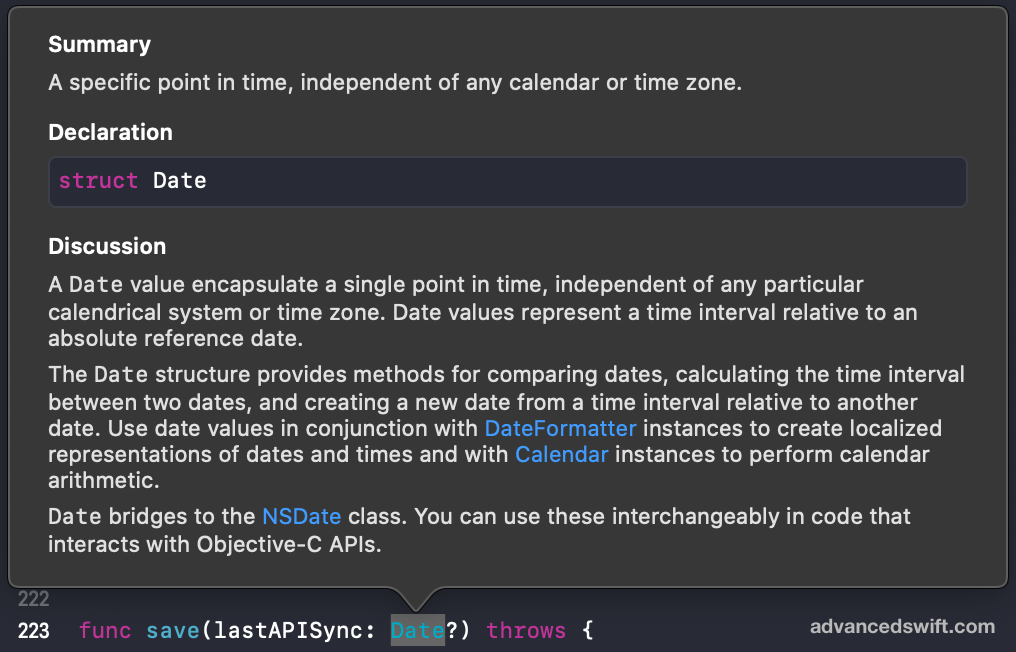
Using Swift markdown comments and Swift Markup allows Xcode to generate Quick Help documentation for your code. For example, by using ///
to add markdown comments and Swift Markup -parameter lastAPISync:
, -throws:
, and -returns:
a Quick Help popup can be generated for the save()
function:
/// Saves changes to the persistent store if the context has uncommitted changes
///
/// - parameter lastAPISync: Date data from the API was last synced.
/// - throws: An error is thrown if unsaved context changes cannot be committed to the persistent store
/// - returns: None
///
///# Notes: #
/// 1. If a lastAPISync Date is provided, the lastAPISync date will be added and saved to the managedObjectContext
/// 2. If there are no unsaved changes and no lastAPISync date is provided, this function does nothing.
///
/// # Example #
/// ```
/// // Save after an API sync
/// let lastAPISync = Date()
/// save(lastAPISync: lastAPISync)
/// // Save local changes
/// save(lastAPISync: nil)
/// ```
func save(lastAPISync: Date?) throws {
if let lastAPISync = lastAPISync {
// ... Save last sync date to Core Data ...
}
if managedObjectContext.hasChanges {
try managedObjectContext.save()
}
}
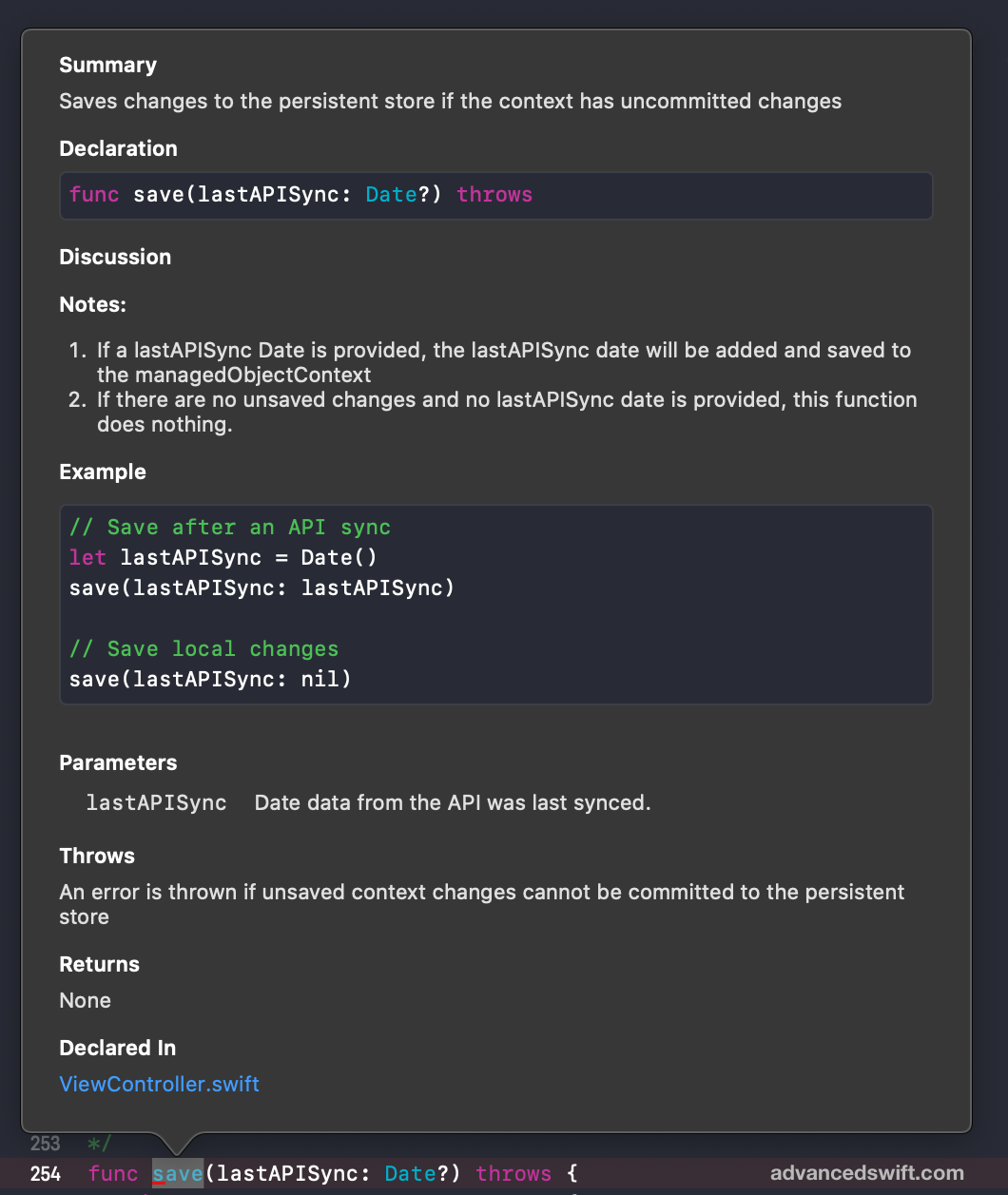
Similarly, the same Quick Help tooltip can also be added by defining markdown comments using /** ... */
:
/**
Saves changes to the persistent store if the context has uncommitted changes
- parameter lastAPISync: Date data from the API was last synced.
- throws: An error is thrown if unsaved context changes cannot be committed to the persistent store
- returns: None
# Notes: #
1. If a lastAPISync Date is provided, the lastAPISync date will be added and saved to the managedObjectContext
2. If there are no unsaved changes and no lastAPISync date is provided, this function does nothing.
# Example #
```
// Save after an API sync
let lastAPISync = Date()
save(lastAPISync: lastAPISync)
// Save local changes
save(lastAPISync: nil)
```
*/
func save(lastAPISync: Date?) throws {
if let lastAPISync = lastAPISync {
// ... Save last sync date to Core Data ...
}
if managedObjectContext.hasChanges {
try managedObjectContext.save()
}
}
Documentation Markup In Swift
That’s it! By using Swift Markup and single line, multi-line, and markdown comments you can document your code and add Xcode Quick Help to your Swift project.